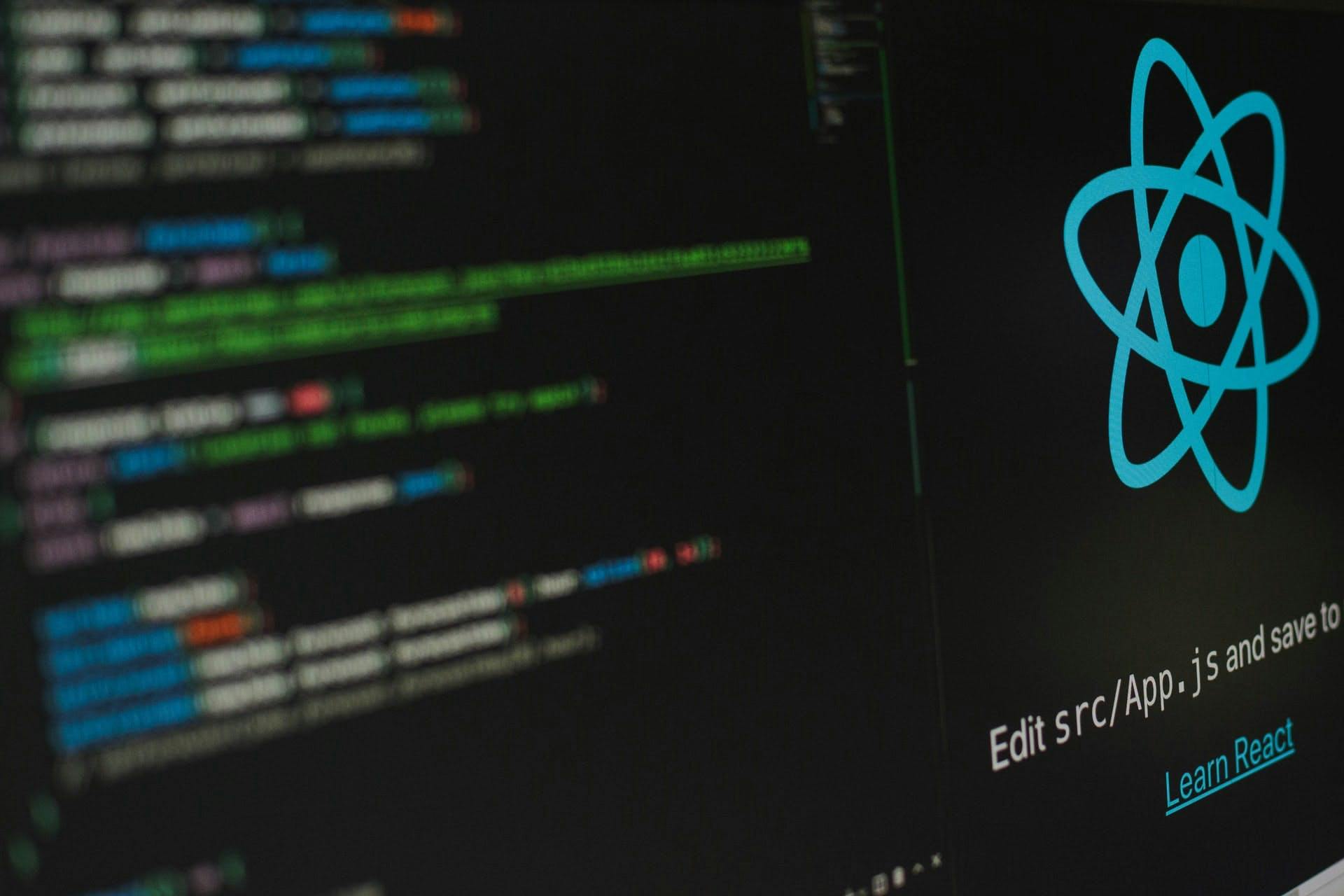
How to add Schema Markup to your Prismic React website
Learn how to set up your schema structured data fields on Prismic so your content writers can have the freedom to manage those on Prismic without a dev's help.
May 10, 2022
1 mins
TABLE OF CONTENTS
Step 1: Set up Prismic
- Within your Custom Type builder, create a new tab called "Schema Markup"
- Add a new Group field called schemaMarkupItems
- Within the group field, add a Rich Text field schemaMarkup - allow multiple paragraphs and only the pre text format
In JSON, the steps above looks like this:
"Schema Markup" : { "schemaMarkupItems" : { "type" : "Group", "config" : { "fields" : { "schemaMarkup" : { "type" : "StructuredText", "config" : { "multi" : "preformatted", "label" : "Schema Markup" } } }, "label" : "Schema Markup" } } }
Using a Group field will allow to content writers to easily include multiple Structured Datas and manage them with ease.
Step 2: Pull the data and render it on your page
Create a base JsonLd component
We refactor this component as we can later utilise it not only for Prismic related schema markup, but also for "static" schemas (eg Organization, Article) which are defined within the codebase.
// Next.js specific component. Use another similar component for other frameworks (eg Helmet for Gatsby) import Head from "next/head"; const JsonLd = ({ children }) => { return ( <Head> <script type="application/ld+json" dangerouslySetInnerHTML={{ __html: JSON.stringify(children) }} /> </Head> ); }; export default JsonLd;
Create a PrismicSchemaMarkup component
We pass the data we get from Prismic and we use the JsonLd component to render it. Several notes worth pointing out:
- We use filter to remove any empty fields within the Group field which might have been added by accident by the content team.
- We use replace to remove any script tags which might have been added by the content team - we add those within the JsonLd component so we don't need them.
- We use the @prismicio/helpers package to check that the field is filled and to convert the richText to a string.
import React from 'react'; import JsonLd from '@components/JsonLd/JsonLd'; import { isFilled, asText } from '@prismicio/helpers'; // Extract the JSON from the Prismic fields and pass it to JsonLd which will render the json within <script application/ld+json/> tags const PrismicSchemaMarkup = ({ data }) => { return ( <> {data ? data .filter(item => isFilled.richText(item.schemaMarkup)) .map((item, i) => { // If included, remove script tags wrappers in order to parse the JSON successfully to the JsonLd component const jsonStr = asText(item.schemaMarkup) .replace(`<script type="application/ld+json">`, '') .replace(`</script>`, ''); return <JsonLd key={`${jsonStr}-${i}`}>{JSON.parse(jsonStr)}</JsonLd>; }) : null} </> ); } export default PrismicSchemaMarkup;
Import it into your page
Remember that this setup might look slightly different depending which React framework you are using.
import PrismicSchemaMarkup from '@/components/PrismicSchemaMarkup'; const LandingPage = ({ doc }) => { return ( <Layout> <PrismicSchemaMarkup data={doc.data.schemaMarkupItems} /> ... </Layout> ); }; export default LandingPage;
Step 3: Generate the Schemas
You content team can now start generating and populating the Schema fields. In order to make this task easier, we created free schema markup generators which can be used to generate the structured data they need and paste it directly into the Prismic fields you created.