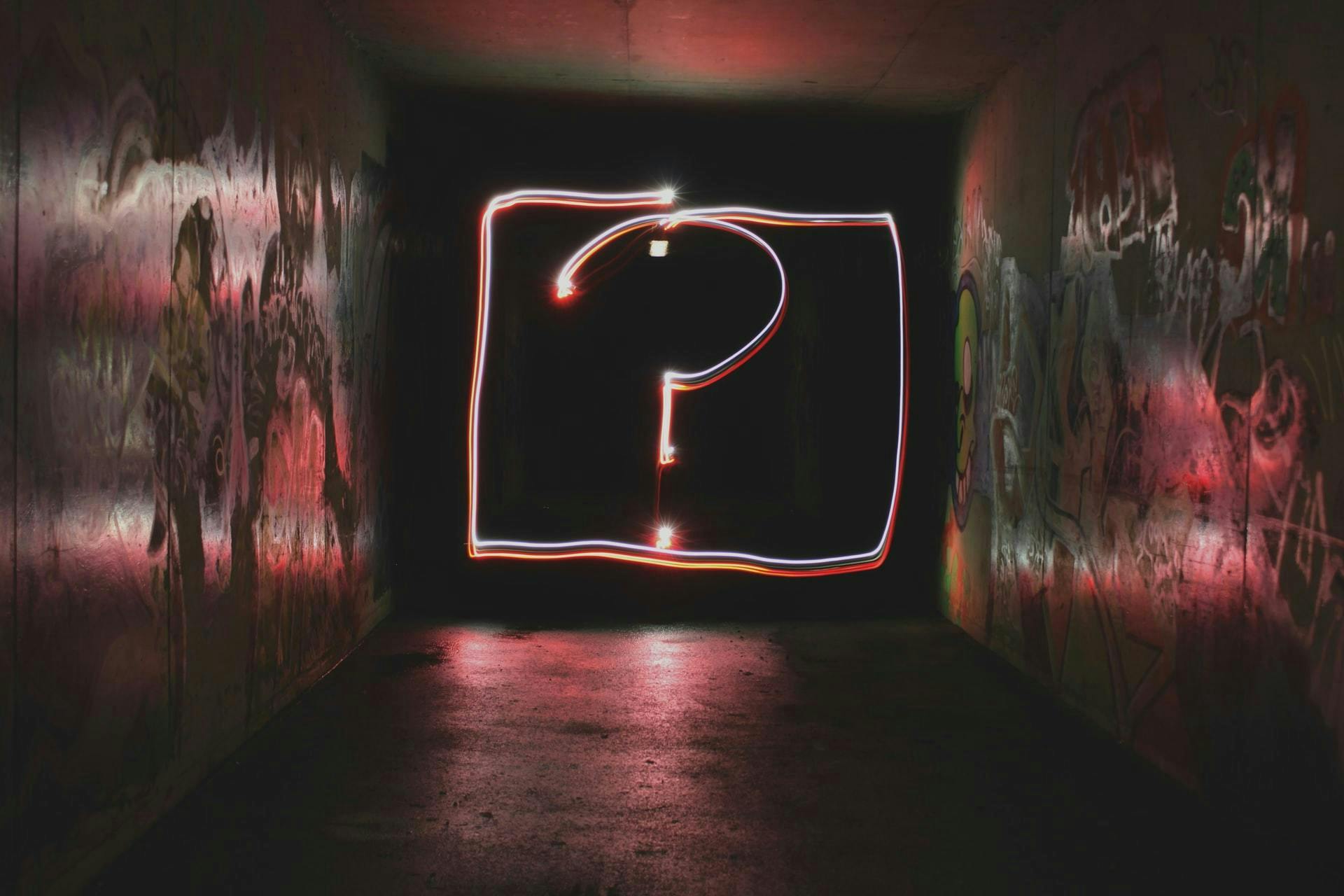
Add FAQ structured data to your articles using Prismic and GatsbyJS
FAQ schema can help your articles get featured in Google's FAQ section. Learn how to add FAQ structured data to your articles in your GatsbyJS application using Prismic fields.
August 10, 2022
3 mins
TABLE OF CONTENTS
What is FAQ structured data?
FAQ structured data is a way to markup your questions and answers for your articles. If your article answers specific questions, it's good to add this markup to your page, as this improves SEO and increases the probability for Google to find them and display them on the search result page.
The FAQ section within Google Result page looks like this

You need to generate JSON containing your questions and answers, they wrap them within a <script type="application/ld+json"> as per the specification provided by Google. Look at the template below to give you an idea of what we will output at the end of this article.
<script type="application/ld+json"> { "@context": "https://schema.org", "@type": "FAQPage", "mainEntity": [{ "@type": "Question", "name": "Question goes here", "acceptedAnswer": { "@type": "Answer", "text": "Answer here" } }, { "@type": "Question", "name": "Question goes here", "acceptedAnswer": { "@type": "Answer", "text": "Answer goes here" } }, { "@type": "Question", "name": "Question goes here", "acceptedAnswer": { "@type": "Answer", "text": "Answer goes here" } }] } </script>
There are several Google guidelines which you should follow.
- Use this FAQ markup if you answer more than one question within your article.
- Avoid adding multiple answers to the same question - Google might think that you are trying to cheat the system.
- Don't add answers which have been submitted by users (for example, comments).
- You shouldn't use the FAQ markup as a marketing/advertising opportunity - it should answer a specific question so avoid any product-specific content.
Step 1: Add FAQ section within your Prismic Article custom type
In your Prismic repository, head over to Custom Types and select the custom type you want to add your FAQ field. In my case, this is the article type.
If you didn't know, you can create Tabs within your documents, in order to organise your content fields. Take advantage of this functionality and add an SEO tab. This is where we will store the FAQ field together with any other SEO related fields (title, description, author, etc)
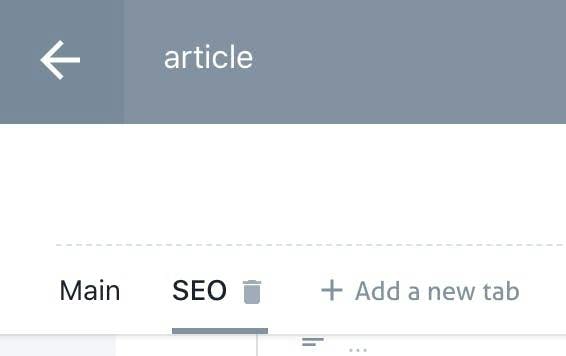
Tabs only affect your organisation within Prismic. Your JSON will stay the same.
Add a Group custom field named faq with a question and answer key text
The custom field should look like this
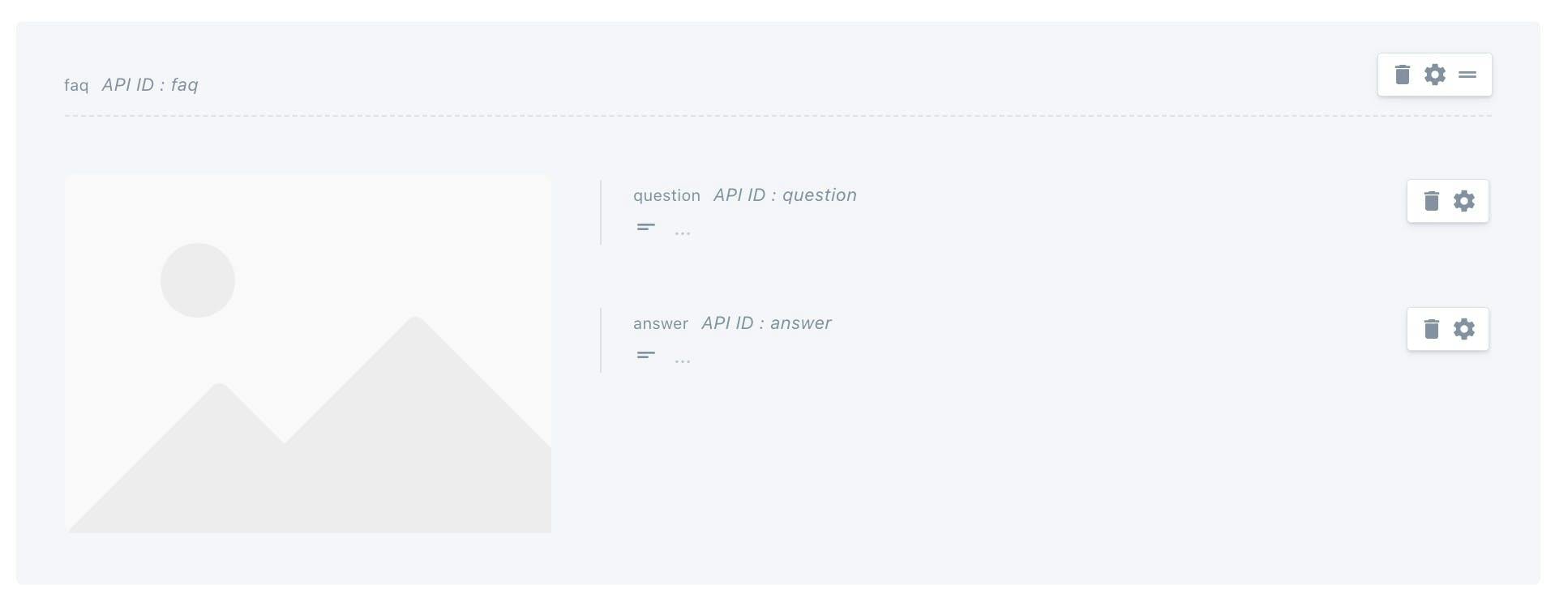
You can now add questions and answers in your articles. Let's add some test data so we can test while we are setting up the code in Gatsby.
You have now completed everything that is needed for Prismic. Next, head over to Gatsby and configure your application to pass the data and create the JSON schema.
Step 2: In Gatsby, retrieve the data and pass it to the SEO component
You need to add the faq group within your GraphQL article query
query ArticleQuery($id: String) { ... faq { answer question } ... }
You can now retrieve the data and assign it to a variable
const article = props.data.prismic.allArticles.edges[0].node const FaqData = article.faq
You should then pass the FaqData to your SEO component as a prop together with your other SEO props.
<SEO ... other SEO related props faqData={FaqData} />
Step 3: Generate the JSON schema and output it using React Helmet
The final step is to generate our JSON by using the template that I showed you above. We will map the faqData prop to match the FAQ schema. Remember to wrap it around an if statement since some articles won't have FAQ data.
let faqSchema = {} if (props.faqData) { const FAQ = props.faqData.map(faq => { return { "@type": "Question", name: faq.question, acceptedAnswer: { "@type": "Answer", text: faq.answer, }, } }) faqSchema = { "@context": "https://schema.org", "@type": "FAQPage", mainEntity: [FAQ], } }
You can then use React Helmet to output the script tag within your head tag. As you are using Gatsby, you should have it installed and configured by default.
In order to ensure that faqSchema is empty, check if it has any keys. If it doesn't, it means that you haven't passed any FAQs from Prismic and therefore, you shouldn't output the script tag.
// components/seo.js ... <Helmet> {Object.keys(faqSchema).length && ( <script type="application/ld+json"> {JSON.stringify(faqSchema)} </script> )} </Helmet>
The result should be in your <head> tag. You can double-check if everything is fine by opening the Dev Tools and inspecting your code.