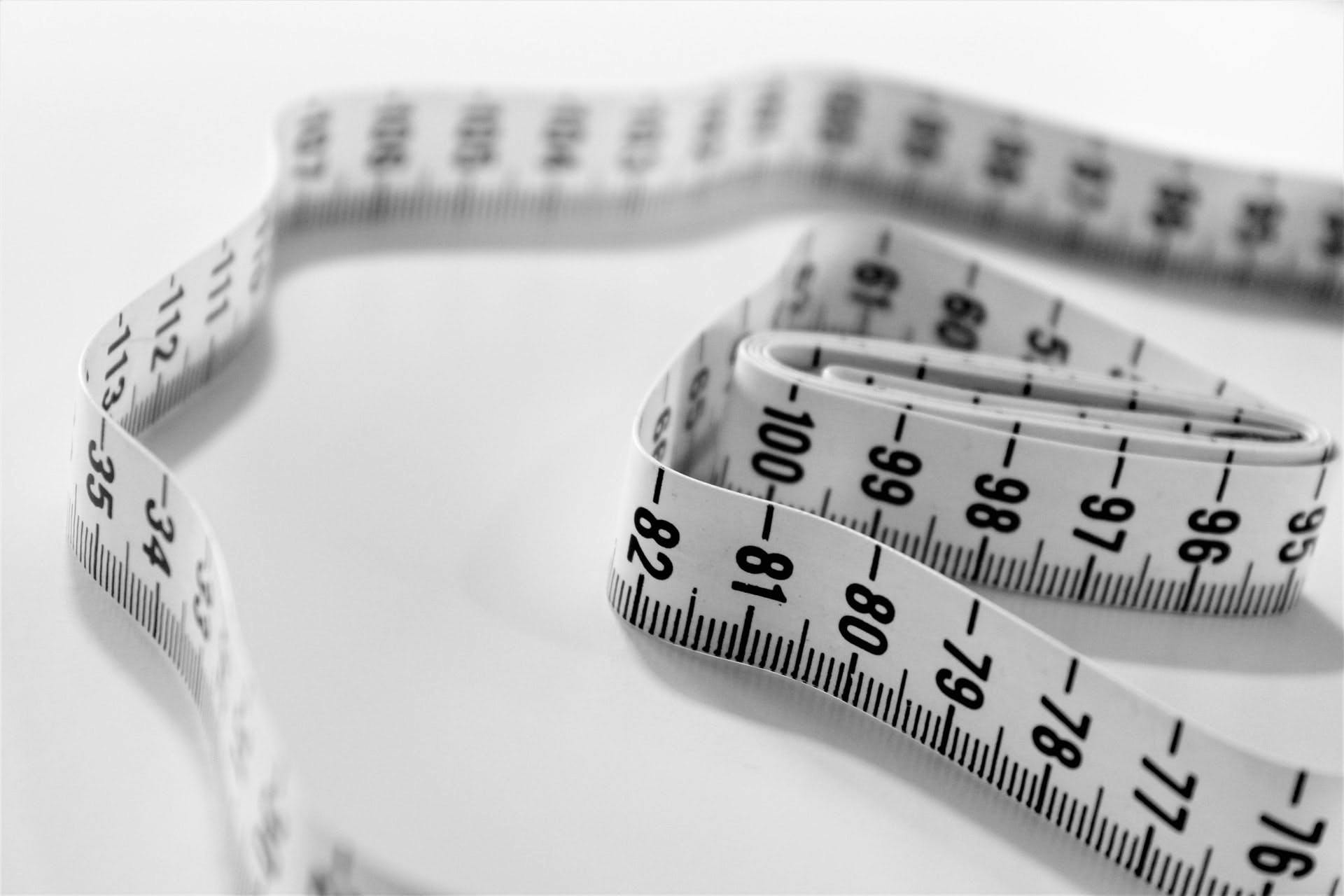
How to query the Prismic API so it to only returns specific fields
A simple way to reduce the JSON size of your Prismic queries (hint: use the fetch parameter)
June 09, 2022
2 mins
TABLE OF CONTENTS
Intro to fetch
Prismic has a less known API option called fetch. It allows you to retrieve only the fields that you need from a custom type.
Use-case example
Personally for me, the most common use-case for fetch is when I only want to query a specific fields for my blog cards.
Create a /utils/fetch.ts file
For my cards I only need to query the image, title and subtitle. If I need the category or the author as well, I can just add a new item to the array.
// https://prismic.io/docs/technologies/rest-api-technical-reference#fetch // Retrieve only specific fields from a model // Helps reduce the JSON size export const contentPostCards: string[] = [ "content-post.image", "content-post.title", "content-post.subtitle", ]
In this file, you can also add other fetch arrays (eg for the author or pricing plans). This way you have these in a centralised place which makes managing you fetch queries easier.
Update your API call
Within you Prismic query, the only thing you need to add is a fetch option with your newly created array
import { contentPostCards } from "utils/fetch" let relatedPosts = [] await client .get({ predicates: [predicate.at("document.type", "content-post"), predicate.similar(doc.id, 500)], orderings: [{ field: "my.content-post.publishedAt", direction: "desc" }], pageSize: 3, fetch: contentPostCards, }) .then(function (response) { relatedPosts = response.results.filter((post) => post.uid !== doc.uid) })
Comparison
Now the array is a lot smaller. It doesn't include things like SEO tags and slices. Let's compare both results:
Not using fetch
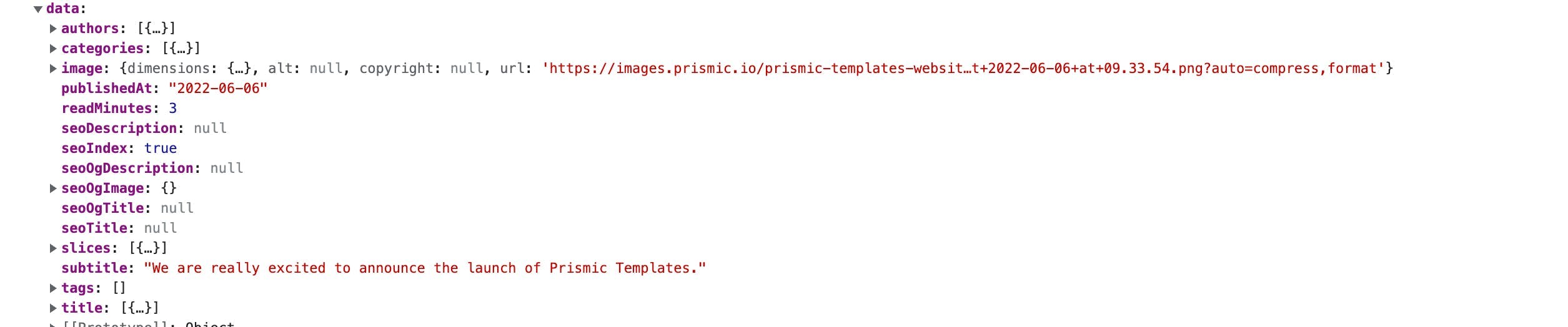
Using fetch
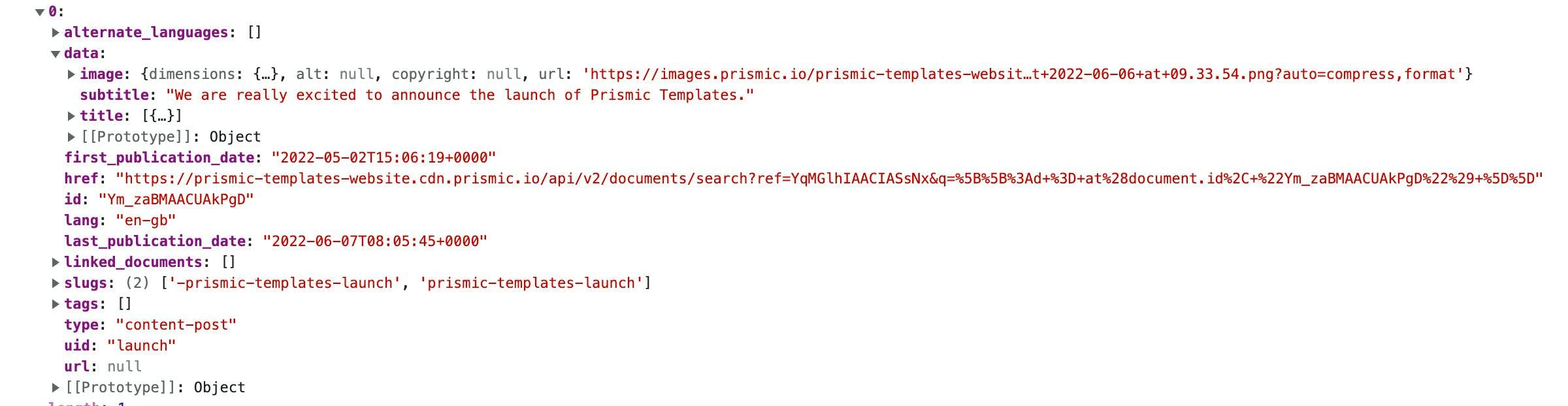
It would make a negligible difference when you only have a couple of cards on the page, but if you are querying 100 articles at the same time, these small differences can become more noticeable on your page size.
An alternative - GraphQuery
It's worth mentioning that Prismic recently released the GraphQuery API, which replaces both fetchLinks and fetch. It allows you to do both selective fetching (what fetch does) and give you an ability to do deep fetching (what fetchLinks does).
However, in simple cases I still revert back to fetchLinks and fetch, as its API is a lot easier to use.
A separate tutorial on how to easily use GraphQuery is coming soon.